Learn it by examples – (LEDs)
By David Kebo Houngninou
On this page, you will find some interfacing experiments using the ARM Cortex M3.
– The evaluation board we target is the MCBSTM32C running on the STM32F107VC microcontroller.
– The tutorial focuses on configuring the MCBSTM32C and interfacing.
– Datasheets and documentation for STMicroelectronics STM32F107VC core is available at: http://www.keil.com/dd/chip/4889.htm.
– The reference used for this tutorial is the RM0008 Reference manual for STM32F107xx advanced ARMĀ®-based 32-bit MCUs.
Tutorial 3: Blinking the LEDs
Blink the LEDs (Read-modify-write method)
1 2 3 4 5 |
const long led_mask[] = {1<<15, 1<<14, 1<<13, 1<<12, 1<<11, 1<<10, 1<<9, 1<<8}; GPIOE->ODR |= led_mask[num]; /*Turn LED num on*/ for (i = 0; i < ((AD_val << 8) + 100000); i++); /*ADC delay*/ GPIOE->ODR &= ~led_mask[num]; /*Turn LED num off*/ |
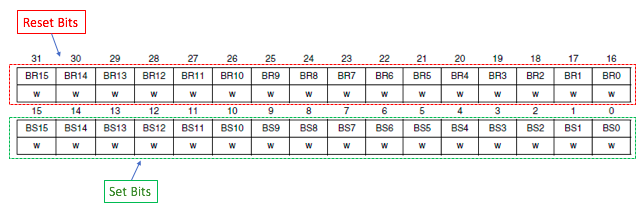
Port output data register
1 |
GPIOE->ODR |= led_mask[num]; /*Turn LED num on*/ |
Blink the LEDs (Atomic method)
1 2 3 4 5 |
const long led_mask[] = {1<<15, 1<<14, 1<<13, 1<<12, 1<<11, 1<<10, 1<<9, 1<<8}; GPIOE->BSRR = led_mask[num]; /*Turn LED num on*/ for (i = 0; i < ((AD_val << 8) + 100000); i++); /*ADC delay*/ GPIOE->BSRR = led_mask[num] << 16; /*Turn LED num off*/ |

Port output data register
Note: Shifting by 16 bits goes from Set bits to Reset bits